![]() | To look at the definition of a pre-existing exercise/example on the site, click the blue </> link on its bottom-right corner. (Seeing these links requires that you are logged in.) To look at the definition of an entire page on the site, click the ![]() |
If you are interested in authoring but don't want to install CS Circles on your own, please contact us for a sandbox account.
This page documents the various shortcodes that are used to describe elements of a CS Circles lesson. The idea of shortcodes is not unique to CS Circles, they come from WordPress. However, we have added a number of new shortcodes to our site.
If you've never used WordPress before, the most important thing to know is that shortcodes extend the language of HTML, and it is in this extended language that page content is written. So any valid HTML will also be valid inside of a CS Circles lesson, for example this lets us use bulleted lists (e.g. see Lesson 0), boldface, italics, tables, images, colored text, headings, etc. The WordPress "Visual Editor" means that you don't have to be an HTML expert to write lessons since it will do this formatting for you in a graphical way where you need it. But some basic knowledge of HTML is very useful.
We start with some basic examples of how shortcodes are like an extension of HTML. Then we go over the ones we think will be most useful in creating lessons. Then we describe the more specialized ones.
![]() | In some cases it is easier to write lessons in HTML mode, which you can access here once your account has editor permission: ![]() |
Warmup: collapsible
and accordion
The collapsible
is a basic example of a UI element; its goal is to provide some information that can be expanded or collapsed. It is analogous to the anchor (a) html tag, which you probably know is written in source as
<a href="http://cscircles.ca">linked text</a>and which causes this to be displayed: linked text
The code to create a collapsible
element is:
[collapsible caption="your caption here"] shown or hidden body [/collapsible]and this results in the following being displayed, which you can click to expand or collapse:
See how clicking opens and closes it?
If you want a collapsible
that is open by default, add the show valueless argument:
[collapsible show caption="..."] ... [/collapsible]Next,
accordion
elements are used to group several collapsible elements so that when one is opened, all others close. It takes no arguments; the most basic example is
[accordion] [collapsible caption="title 1"] body 1 [/collapsible] [collapsible caption="title 2"] body 2 [/collapsible] [/accordion]This generates:
Note that if body 1 is open, clicking on title 2 both opens body 2 and closes body 1.
Shortcodes for Python: pyExample
, escaping, and @file: references
The pyExample
shortcode lets you show a small example program. You specify the code, a title, and optionally a description. For instance,
[pyExample code="print(2*2)" title="Hip to be square"]Optional description[/pyExample]will produce
There are numerous additional options for pyExample
, in fact they are the same as for pyBox
, but we talk about these options later on. Instead, let us mention escaping in shortcodes. For example, what if you want your example code
argument to contain double-quotes? There are a few rules you should know and that you can take advantage of:
- the following applies to shortcode arguments, but not shortcode bodies
- you can use single quotes to contain arguments instead of double quotes
- you can use backslashes to escape either quote, newlines, and backslashes
- real newlines (pressing Enter) also work, but not several in a row (due to WordPress editor limitations)
For example, mixing a few of these:
[pyExample code='print(1)
print(\'four\')\nprint("9")' title="It's hip to be square"] [/pyExample]This gives:
Sometimes it is awkward to correctly type out a chunk of code with lots of escaping. For this reason you also can use an @file: reference. This tells Python to go fetch a file from the file system (in the wp-content/lesson_files
folder) and use it as the value of that argument; no escaping is needed within that file. An example in Lesson 2 is:
[pyExample code="@file:lesson2/printy.py"] [/pyExample]which generates
You can see the actual contents of the printy.py
file here.
Let us mention that the body of a shortcode doesn't need escaping. The body of a shortcode can contain more shortcodes, but shortcode arguments can't contain shortcodes (this is more or less analogous to HTML).
The remaining sections are shown below in an accordion. You do not have to read them in order.
pyBox
(& pyScramble
)
The pyBox
shortcode is used to define exercises in our system. It can be used in many different ways and accordingly, it has a lot of different options. We'll mostly describe the elements by example.
Basics: input
/stdin, solver
(model solution), body
[pyBox input1='2' input2='3' solver='print(-int(input()))'] Read an integer from input and print its negative. [/pyBox]
If you only have one test case, you can write input
instead of input1
.
Generating random test cases: generator
(and repeats
)
[pyBox generator="print(_rint(10, 100))" repeats=3 solver='print(-int(input()))'] [/pyBox]
Here, _rint
is a short-form for Python's random.randint
function, defined here in a utilities file.
Evaluating a student-defined function: autotests
Whereas the previous examples give students input through stdin and check their output through stdout, the second testing paradigm is to make them define functions. Then the exact type and value of the function's return value of the student's code is compared to that of the solver code.
[pyBox solver="def square(x): return x*x" autotests="square(3)\nsquare(4)"] Define a functionsquare(x)
that takes a numberx
and returns its square. [/pyBox]
To do this with repetition and randomization, use this construction instead:
autotests="for i in range(3):\n square(_rint(1, 100))"
Pre-defining user variables/functions: precode
You can define a utility function for the user (see 11c.rightperimeter for an example), or use a variable as a way of providing test input (see 1.heads for an example).
[pyBox solver="print(mynum*mynum)" precode="mynum=_rint(1, 100)" repeats=2] The grader will define a variable <code>mynum</code> for you. Print out its square.[/pyBox]
Testing user variables: autotests
The autotests
argument not only can be used to call functions (like above) but it also can be used to test variables. This is often used with precode
, see for example 1.swap.
[pyBox autotests="x" solver="x=5"]Define a variable x
equal to the number five.[/pyBox]
Default Code (code
) and Code Scrambles: pyScramble
To create a Code Scramble exercise, use pyScramble instead of pyBox:
[pyScramble solver="a=1\nb=a+1\nc=b+1"] Make this not crash.[/pyScramble]
The solver
code is randomly permuted initially. A better option is to use code
(or its synonym defaultcode
) to start the student in some well-scrambled state.
Note: you also can use defaultcode
in non-scrambled pyBox
exercises if you want to give the student a template, or a buggy program to debug.
Other useful pyBox arguments
title
: Gives a title at the top of the exercise. Mandatory for exercises when published.slug
: A permanent unique identifier like 1.swap or 11c.rightperimeter. Mandatory for exercises when published. Use only letters, numbers and periods. It is not user-facing but is needed for internal use; even if you change some problem options, as long as the slug stays the same the history will be remembered by the system.taboo
: comma-separated list of keywords (like "for, while", space optional) which can not appear in the submitted code. Each keyword optionally is of the form display|regex where regex is the regex to search for and display is what to display to the user. E.g., taboo="lower|\\.lower" would say "lower is not allowed" but searches for regex "*\.lower"desirederror
: (e.g. lesson 6) use this when you want the student to create an error. The last line of stderr is compared with the string passed asdesirederror
. The exercise is completed successfully if they match. See an example here.grader
: By default, when there is output to be compared, exercises use a grader that compares the output to see if it is exactly equal to the expected one. To override this,grader
should be defined equal to a chunk of Python code which (a) takes string array variables InputLines, ExpectedLines, and OutputLines, (b) prints out Y if the output is deemed correct and N otherwise, and (c) prints out any additional feedback (typically only for incorrect cases) after that first character. See one of several predefined graders for examples: @file:anyorder.py, @file:real-per-line.py, and @file:warmup.py.generator
: Python code that prints procedurally-generated stdin. See 9.abs for an example.
maxeditdistance
: Enforces a maximum number of changed characters from the original program. See 1e.joe for an example.
pyShort
, pyMulti
, pyMultiScramble
These are short-answer exercises, multiple-choice exercises, and unscrambling exercises. All three accept an
epilogue
option which is a string (or file reference) to give an extra message once the problem is completed correctly. They should have a title
and slug
the same as pyBox
does.
Short Answer: pyShort
[pyShort answer="Q"]what is the letter after P?[/pyShort]
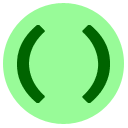
Arguments:
answer
: the correct answertype
(optional): put "number" to treat "5.00" the same as "+5" etc. If left out, string comparison is used. See 1.plusminus for example.
Multiple Choice: pyMulti
[pyMulti right="correct answer" wrong="bad1\nbad2\n..."]Body[/pyMulti]
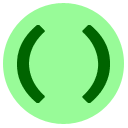
Descrambling (without code): pyMultiScramble
[pyMultiScramble answer="put this first\nsecond\nthird\n4"] content [/pyMultiScramble]
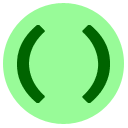
- third
- 4
- second
- put this first
See an example at 12.ordering.
pyHint
, pyLink
, pyWarn
, latex
pyWarn
Gives a warning.
[pyWarn] Warning text [/pyWarn]
![]() | Warning text |
pyHint
Gives a clickable pop-up hint (also consider using collapsible hints).
Here's a [pyHint hint="the hint text"]hint[/pyHint]!Here's a hint!
pyLink
Gives a clickable link to code in the console.
This [pyLink code='print("|"*10)']link[/pyLink] rules.This link rules.
Note: often we use an @file: reference for the code
parameter.
latex (WP-latex plugin)
br
, pyRaw
, style
- Despite significant effort, we are unable to get newlines to work well inside of shortcode bodies. This has to do with WordPress' "autop" system that automagically determines where to put <p> and </p> tags. To compensate for this, we define the shortcode
which forces an html<br>
newline to occur. - If you need specific html that the visual editor mangles, use
@file:filename
which will put the contents of the specified file at that location, after all other processing on the page is done. We use this in lessons 6, 8, and 9. - If you want specific css styles on your page, use the
style
shortcode. For example,<h6>Ta-da</h6>
createsTa-da
authorship
, pyRecall
, youtube
, pyVis
- Write to indicate the authorship history of a lesson. Look at the source code of any page for an example.
- Use
pyRecall
for translation. See more information on the Translation Notes page. - Use
youtube
to embed a youtube video. See lesson 7a for an example. - Use
pyVis
to do an embedded visualization. For example[pyVis code='x = 2\nx += 1']
The visualization will be cached unless the word "random" appears in the source code.
Help: for help with authoring problems, please create a new issue at https://github.com/cemc/cscircles-wp-content/issues