A computer program looks like a code language, which is necessary for the computer to precisely understand what your commands mean. But, being a code language makes it harder for humans to read. To compensate for this, you are allowed to write extra notes in your program that the computer ignores. These notes are called comments.
In Python, any line of instructions containing the #
symbol ("pound sign" or "hash") denotes the start of a comment. The rest of the line will be ignored when the program is run. Here is an example.
Because the second line started with a #
sign, Python totally ignored that line, and as you can see, the number 2
was not printed. Common uses for comments include:
- explaining parts of the program, for you or other people to read later;
- leaving "to do" notes, when you write a longer program;
- temporarily disabling ("commenting out") a line of a program without totally deleting it, so that it is easier to put back in later.
Here is an exercise to illustrate. If you edit the code too much and want to bring back the default version of the code, select Reset code to default.
Strings
Strings are sequences of letters and numbers, or in other words, chunks of text. They are surrounded by two quotes for protection: for example in Lesson 0 the part "Hello, World!"
of the first program was a string. If a pound sign #
appears in a string, then it does not get treated as a comment:
This behaviour is because the part inside the quotes ""
is a string literal, meaning that it should be literally copied and not interpreted as a command. Similarly, print("3 + 4")
will not print the number 7, but just the string 3 + 4
.
Escape Sequences
What if you want to include the quote character "
inside of a string? If you try to execute print("I said "Wow!" to him")
this causes an error: the problem is that Python sees one string "I said "
followed by something Wow!
which is not in the string. This is not what we intended!
Python does have two simple ways to put quote symbols in strings.
- You are allowed to start and end a string literal with single quotes (also known as apostrophes), like
'blah blah'
. Then, double quotes can go in between, such as'I said "Wow!" to him.'
- You can put a backslash character followed by a quote (
\"
or\'
). This is called an escape sequence and Python will remove the backslash, and put just the quote in the string. Here is an example.
Furthermore, because of escape sequences, backslash (\
) is a special character. So to include a backslash in a string, you actually need to "escape it" with a second backslash, or in other words you need to write \\
in the string literal.
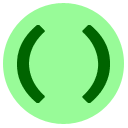
print("Backslashes \\ and single quotes \' and double quotes \" and pound signs # are awesome!")
There are other escape sequences, like "newline," that we won't discuss right now. For now, you are ready to move on to the next lesson!