This lesson will allow you to do complex case-checking more elegantly, using two new parts of the Python language.
- after an
if «C»
statement, anelse
statement executes only ifC
is false - you can combine boolean conditions using
A and B
,A or B
, andnot A
else
A common task in writing programs is that you want to test some condition, and take either one action or another action, depending on the condition is true or false. Earlier, an exercise asked you to do something if age
was less than 0, and something else if age
is not less than 0; the code was something like
if age >= 0: print('You are not necessarily a time traveller') if age < 0: print('You are a time traveller')Now let's rewrite it to use
else
.
More generally, you use else
via the following pair of block statements:
if «test»: «true-body» # an indented block else: «false-body» # another indented blockPython evaluates the test. If it is true the true-body is executed, and if it is false the false-body is executed.
The Philosophy of else
We don't get new powers from else
, but it makes code much easier to read, debug, and maintain. Here are two code fragments that do the same thing:
Version A
if height < 256: print('Too short for this ride') else: print('Welcome aboard!') |
Version B
if height < 256: print('Too short for this ride') if height >= 256: print('Welcome aboard!') |
Both do the same thing, and B doesn't even need else
. However, most programmers would agree that A is better. For example, with A, changing the condition (e.g., using 128 instead of 256) requires only one change to the code instead of two. Also, A can be immediately understood by a human reader, while in B you need to check and think whether or not both, or neither conditions can be true.
![]() | Python has a built-in function abs(x) to compute the absolute value of x . The grader above prevents you from using it, but you can use it later. |
elif
Python introduces one other keyword, elif
, to make it easier to check several conditions in a row. The most basic form of elif
is the following:
if «test1»: «body1» # runs if test1 is true elif «test2»: «body2» # runs if test1 is false and test2 is trueAs you may have guessed,
elif
is short for "else if", since it is the same as putting an if statement inside an else block. But, it leads to shorter code and less indentation, which makes your program easier to read, debug, and edit. What's more, you can combine any number of elif statements in a row and even add an optional else
statement to the end:
if «test1»: «body1» # runs if test1 is true elif «test2»: «body2» # runs if test1 is false and test2 is true elif «test3»: «body3» # runs if test1 & test2 both false and test3 is true else: # these last two lines are optional «else-body» # runs if ALL the tests are false
Here is an example of elif
used inside of a loop. Can you predict the output before it runs?
Boolean Operators: and
, or
, not
You can combine boolean expressions using "and
", "or
", and "not
", which are the same as in the English language.
- The expression
A and B
is true if bothA
is true andB
is true, and false if either is false. (For example, you get wet if it rainsand
you forgot your umbrella.) - The expression
A or B
is true if eitherA
is true orB
is true, and false if both are false. (For example, school is closed if it is a holidayor
it is a weekend.) - The expression
not A
is true ifA
is false, and false ifA
is true. (For example, you are hungry if you havenot
eaten lunch.)
Here is a program which automatically displays all of the possibilities. Just like the 'multiplication tables' you remember from grade school, this is called a truth table.
As an example of using and
, here is a program which converts numbers to letters, with some error-checking.
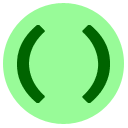
A or B
?not (A or B)
is only true when both A
and B
are false. Also, note that (not A) and (not B)
is only true if both not A
and not B
are true, i.e. if both A
and B
are false. So we have the following equality:(not A) and (not B) = not (A or B)Put a
not
around both sides, so we deducenot ((not A) and (not B)) = not (not (A or B))and observe that
not(not X))
always equals X
, sonot ((not A) and (not B)) = not (not (A or B)) = A or BThis way of rewriting a boolean expression is one of De Morgan's laws.
Order of Operations
Boolean operators have an "order of operations" just like mathematical operators. The order is
NAO: not (highest precedence), and, or (lowest precedence)
so for example,
not x or y and z
means (not x) or (y and z)
We conclude the lesson with a short question using these facts.
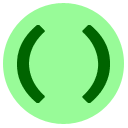
A or not B and Cif (A, B, C) = (False, True, True)? Hint
A or ((not B) and C)substituting the values, we have
False or ((not True) and True)and now simplifying one step at a time gives
False or ((not True) and True)
= False or (False and True)
= False or False
= False