We have seen one function already, print()
, which outputs a message. To use a function you always write its name, followed by some arguments in parentheses ()
. The word argument basically means an input to the function. Then, the function does some action depending on its arguments. When there are multiple arguments to a function, you separate them with commas (,
). For example, you can give multiple arguments to print
; it will print all of them in order, with spaces separating them. We demonstrate in the example below.
![]() | The extra spaces in the sample program above had no effect on the output. Extra spaces are meaningless in most other situations too. However, be careful that extra space at the beginning of a line, called indenting, has a special meaning that can cause errors (click for an example) if used incorrectly. You will see correct indenting a few lessons later. |
A function may also give back a value (like an output). For example the function max()
(short for maximum) gives back the largest out of all of its arguments, which must be numbers.
max(42, 17)
we say that "the function max
returned the value 42
."
The max
function has a friend which behaves similarly: the min
function returns the minimum (smallest) of its arguments.
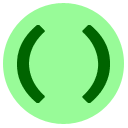
x = 13
y = 7
a = max(x+y, x*2)
b = min(x, y)
print(a,b)
Functions can be combined to create more complicated expressions.
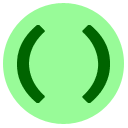
x = min(max(13, 7), 9)
print(x)
You are not limited to using functions that are pre-defined in Python. In a few lessons you will learn how to define new functions!
Common Errors
If you call a function with not enough arguments (inputs) or too many arguments, you get an error. For example, max
requires at least one input:
It's very important to carefully read the errors that you get back, when your code doesn't work. Python will usually give you helpful feedback on what went wrong. However, sometimes you need to look around a little bit to diagnose the problem — here's an example.
Python says there is a syntax error, which means it can't understand what you're trying to do:
Traceback (most recent call last): In line 2 of the code you submitted: bigger = max(3, 4) ^ SyntaxError: invalid syntaxHowever, the line
bigger = max(3, 4)
is actually fine. The problem actually spilled over from the previous line: we forgot to add the closing parenthesis )
after smaller = min(14, 99
and Python started looking at the next line for the )
. So, check the lines before and after what Python suggests, if you are stuck debugging your programs.
Exercise
This is a two-part exercise using the min
and max
functions. There are connections between the cities of Maxime and Miniac with several bridges. There is a separate limit on the amount of weight that can be transported across each bridge.
Exercise: Code Scramble
Here is another code scramble, where you must drag-and-drop the lines to rearrange them into a correct program.
Once you finish the exercise above, you have two choices: