Lesson 7 has three parts A, B, C which can be completed in any order.
So far, we have performed math calculations using Python's operators +
, -
, *
, /
and the functions max
and min
. In this lesson we will see some more operators and functions and learn how to perform more complex calculations.
Math Operators
We have already seen how to use operators for addition (a + b
), subtraction (a - b
), multiplication (a * b
) and division (a / b
). We will now learn about three additional operators.
- The power operator
a ** b
computesab
(a
multiplied by itselfb
times). For example,2 ** 3
produces8
(which is 2×2×2). - The integer division operator
a // b
computes the "quotient" ofa
divided byb
and ignores the remainder. For example,14 // 3
produces4
. - The modulus operator
a % b
computes the remainder whena
is divided byb
. For example,14 % 3
produces2
.
The modulus operator is used for a variety of tasks. It can be used to answer questions like these ones:
- If the time now is 10 o'clock, what will be the time 100 hours from now? (requires modulus by 12)
- Will the year 2032 be a leap year? (requires modulus by 4, 100, and 400)
Checking leap years is an example of divisibility testing; in the next exercise we ask you to write a program that performs divisibility testing in general.
Math Functions
Python can compute most of the mathematical functions found on a scientific calculator.
sqrt(x)
computes the square root of the numberx
.exp(x)
andlog(x)
are the exponential and natural logarithmic functions.sin(x)
,cos(x)
,tan(x)
and other trigonometric functions are available.pi
, the mathematical constant3.1415...
, is also included.
![]() | When using Python's trigonometric functions, the angle x must be expressed in radians, not degrees. |
Python includes such a large number of functions that they are organized into groups called modules. The above functions belong to the math
module. Before using any functions from a module, you must import the module as shown in the example below. To use a function from a module you must type the module name, followed by a period, followed by the name of the function.
Putting it all together
As you saw in the previous exercise, you can build mathematical expressions by combining operators. Python evaluates the operators using the same "order of operations" that we learn about in math class:
Brackets first, then Exponents, followed by Division and Multiplication, then finally Addition and Subtraction,
which we remember by the acronym "BEDMAS". Integer division and modulus fit into the "Division and Multiplication" category. For example, the expression
3 * (1 + 2) ** 2 % 4is evaluated by performing the addition in brackets (1+2 = 3), then the exponent (3 ** 2 = 9) , then the multiplication (3 * 9 = 27), and finally the modulus, producing a final result of 27 % 4 =
3
.
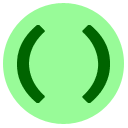
6 - 52 // 5 ** 2
Integer division with negative numbers: The expressions a // b
and int(a / b)
are the same when a
and b
are positive. However, when a
is negative, a // b
uses "round towards negative infinity" and int(a / b)
uses "round towards zero."
Integers and Floating-Point Numbers
The result of a mathematical expression is a number. As we saw previously, each number is stored as one of the two possible types: int
or float
. The int
type represents integers, both positive and negative, that can be as big as you want.
![]() | Python does not accept numbers written in the form 1 000 000 or 1,000,000 . Type 1000000 instead. |
The float
type represents decimal numbers. Just as a simple calculator stores 1/3
as its approximate value 0.33333333
, Python also stores decimal numbers as their approximate values.
![]() | Because Python uses approximations of decimal numbers, certain equations which are mathematically true may not be true in Python.
For this reason, it is important to allow some tolerance for these approximations when comparing numbers of type float . For example, in the internal grader used by this website, any float output is marked as correct if it is approximately equal to the expected answer. |
We finish this lesson with some exercises.
Congratulations! After completing these exercises you are ready to move to another lesson.