Up to now, all of our programs have executed their instructions in order without skipping any lines. Here's an example; step back and forth through the code with the buttons or your keyboard's arrow keys.
The new ability in this lesson is the if statement, which allows us to perform actions only when certain conditions are met, and skip lines otherwise. For example, let's change our program to output an extra message when someone's age is large enough. We will add two lines: if age > 65:
(meaning, "if age
is greater than 65") and print('Enjoy retirement!')
. Now step through the code again:
Notice that the new print statement was skipped because age
was only 31! What happens with the exact same program when birthYear
is changed to 1928?
Do you notice the difference? The bottom version (with age
85) printed out two lines and did not skip any. This is exactly the purpose of the if statement: to check something (is
age
greater than 65?) and to perform certain actions (printing Enjoy retirement!
) only when the check holds true.
We call the thing being checked the condition, and the statements that are dependent on whether the condition holds true the body. The body has to be indented which means there are extra spaces at the start of the line (like in the example above). The body can actually contain more than one statement: to do this, write several indented lines. Here's an example.
- Try running this code in the console or visualizer with birth year 1982. Both of the indented lines are skipped.
In the next exercise, we ask you to write a short program with boolean comparisons. We already saw in the examples above that "if x > y:
" means "if x
is greater than y
". You might also find the following operations useful:
if x < y:
means "if x is less than y"if x >= y:
means "if x is greater than or equal to y"if x <= y:
means "if x is less than or equal to y"
Structure of an If Statement
- The first line,
if «condition»:
has three parts: the wordif
, the«condition»
which must be a True/False expression (more on this below), and a colon:
- Then, the body consisting of one or more indented lines. The number of spaces (the amount of indentation) doesn't matter, but being inconsistent will cause an error since Python uses the amount of indentation to determine where you mean the body to start or stop.
The body of an if statement is an example of a programming "block". Blocks will be used in many other places later on, including "for loops" and defining your own functions. (Certain other programming languages indicate blocks is with curly braces
.){}
The next exercise is actually 4 exercises in one. You will arrange the lines of a program to get several different results. Click on the horizontal bars to open each part; you can do them in any order. As usual, in each part, drag and drop the lines of code to rearrange them.
True, False, and bool
So far, in the «condition»
part of an if
statement, we saw a simple numerical comparison x > y
, which is true when x
is greater than y
and false when x
is less than or equal to y
. More generally, any true/false value is called Boolean (see George Boole). In Python, the bool
type is used to represent Boolean values; only two bool
values exist, True
and False
.
Note that in Python, when you use the bool
values True
and False
directly in a program, they must be capitalized or you will get an error.
Boolean comparisons
The operators >
, <
, <=
, and >=
compare two numbers and give back a bool. There are two other ways to compare numbers:
x == y
is the equality operator, it returnsTrue
ifx
andy
are equalx != y
is the not-equal operator, it returnsTrue
ifx
andy
are not equal==
and!=
also work for strings and other types of data
(There are two equal signs ==
here since the single equals sign already has the meaning in x = «expression»
of "assign the variable x
the value of «expression». Confusing =
with ==
is a common source of bugs.)
Here is an exercise that will involve one of the new comparisons.
We just introduced the concept of a block (several lines grouped together at the same indentation level). You can have a block inside another block:
if password=='openSesame': print('User logged on.') if hour>17: print('Good evening!') print('Enter a command:')Here the outer block consists of 4 lines and the inner block consists of just 1 line:
if password=='openSesame':print('User logged on.')
if hour>17:print('Good evening!')print('Enter a command:')
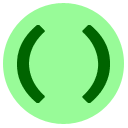
if
shour>17
is false (and the inner block is skipped), the line print('Enter a command:')
of the outer block will still be executed.Now, you must use nested blocks to write a slightly more complex age calculator.
Later, in lesson 9, we will see that situations involving multiple boolean checks can be simplified using the else
and elif
(else if) commands as well as the Boolean operators "and", "or", and "not". For now, you are ready to proceed to the next lesson.