Variables act as "storage locations" for data in a program. They are a way of naming information for later usage. Each variable has a name; an example variable name we will use is myLuckyNumber
. To store information in a variable, we write a command using an equal sign in the following way:
«the variable name» = «the value you want to store»(We use «double angle brackets» in our lessons, like above, to indicate special parts of expressions.) For example, the Python line
myLuckyNumber = 13stores the value
13
in the variable myLuckyNumber
. Then, anywhere you write the variable name myLuckyNumber
again, Python retrieves the stored value. Below, there is a short example of using variables. It has more than one line of instructions: Python executes the first line, then the second line, and so forth until it reaches the last line. Press the Run program button to see what it does.
Look at the 5 lines of the program in order, and how they correspond to the output. As you can see, myLuckyNumber
keeps its value of 13
for the first two print
statements, then its value is changed to 7
. We also introduced the plus operator (+
) above, which adds two numbers together. Similarly, there are operators for subtraction (-
), multiplication (*
), and division (/
). We'll return to these in a later lesson. You can simulate the memory storage of a computer with paper and pencil, by keeping track of the values in a table. Here is an example; remember that *
means multiplication in Python.
first = 2 second = 3 third = first * second second = third - first first = first + second + third third = second * firstIdea: We use a table to keep track of the values as they change. Scroll to the bottom to see the final answer.
Statement | Values after statement executes | ||
---|---|---|---|
first | second | third |
first = 2 | 2 | ||
second = 3 | 2 | 3 | |
third = first * second | 2 | 3 | 6 |
second = third - first | 2 | 3 4 | 6 |
first = first + second + third | 2 12 | 4 | 6 |
third = second * first | 12 | 4 | 6 48 |
Thus at the end of the program, the value of first
is 12
, the value of second
is 4
, and the value of third
is 48
.
Drawing a table like this on pencil and paper is always a good idea and helpful when understanding or fixing code. We also have an automated Python3 visualization tool to virtually execute programs like this one step at a time (see also the link in the top menu). Here's what it looks like when we run the same program on the visualizer. Use the Forward > button or press the arrow key on your keyboard to step forward (or back). Note how the variables change as each line executes. Here is a short answer exercise about variables.
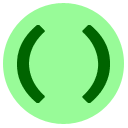
x
Marks the Spotx
after these commands execute?
x = 10
x = x + x
x = x - 5
Two Common Errors
If you ask Python about a variable that has not been defined, you get an error.
As you can see, we get an error message sayingNameError: name 'trouble' is not defined
. Sometimes you can get errors like this from simple typos: if you define a variable address=32
, then try to print(adress)
, the same type of error occurs. Another error has to do with accidentally swapping the sides of an =
statement.
The first line is fine but the second line causes an error: Python thinks the second line 4 = x
is trying to change the value of 4
, but you are only allowed to change the values of variables, and 4
is not a variable. While A = B
and B = A
are the same in mathematics, they are different in programming.
Exercise
This is a warm-up to get you started with variables.
Code Scramble
The next item in this lesson is a new type of programming exercise, where you don't have to do any programming. We will provide you with a correct program, but the catch is that its lines have been put into a scrambled-up order. Your job is to drag-and-drop the lines to rearrange them into a correct program.
Exchange Program
Here is the last exercise in this lesson.
Once you get this exercise correct, you are ready to move on to the next lesson. Click the Next button below.